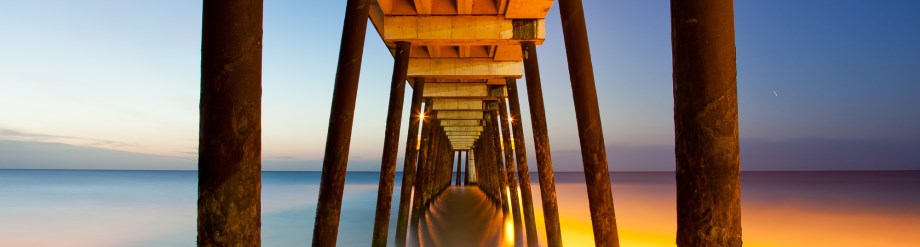
Using Laravel and Guzzle 6 to post to a Google Forms Spreadsheet
<p><a href="http://www.google.com/forms/about/">Google Forms</a> is a nice tool. Anyone who's not able to code can, in minutes, create a form to manage registrations, RSVPs, surveys, polls, and collect any kind of data. You put some elements on a form, get a link, send to your friends and every time they click send, bam!, data is stored in a nice and powerful <a href="https://docs.google.com/spreadsheets/">Google Spreadsheet</a>. Google Forms design is... nice, yeah, maybe not really. There are a bunch of themes available, but you cannot really style it your own way, at least not from Google's user interface, so you always get one of those:</p> <p><img src="http://i.imgur.com/qViEki8.png" alt="Image" /></p> <p>So, to build something different, like this:</p> <p><img src="http://i.imgur.com/iytdjL8.png" alt="Image" /></p> <p><a href="http://morning.am/tutorials/how-to-style-google-forms/">You can grab the whole form tag and paste it on your page</a>, but then there's the confirmation page, shown every time people hit send:</p> <p><img src="http://i.imgur.com/OIDRCjf.png" alt="Image" /></p> <p>You would have to have some Javascript to change it. Not cool, right?</p> <p>In PHP it's easy to post to it and give your user a custom feedback, here's a the code using Laravel and Guzzle, directly from a Laravel route:</p> <pre class="prettyprint"><code >Route::post('googleforms', function () { $client = new GuzzleHttp\Client(); $formId = 'ABCDXRn7hdsLhrlDxalvDG6F4ISHQbT4duG2lZZl6OAHY'; $data = [ 'form_params' => [ 'entry.123458658' => Input::get('name'), 'entry.123450697' => Input::get('city'), 'entry.123453423' => Input::get('school'), 'entry.1234596279' => Input::get('email'), 'entry.123452185' => Input::get('phone'), ] ]; $client->post("https://docs.google.com/forms/d/$formId/formResponse", $data); return view('formSent'); }); </code></pre> <p>You just need your Google Form ID:</p> <p><img src="http://i.imgur.com/b3wwLgL.png" alt="Google Form ID" /></p> <p>And the names of the inputs to post to, which you can grab looking at the HTML souce of your Google Form.</p> <p>Here's a class which does the same:</p> <pre class="prettyprint"><code >use GuzzleHttp\Client as Guzzle; class GoogleForm { protected $formId; protected $httpClient; public function __construct($formId = null, Guzzle $httpClient) { $this->httpClient = $httpClient; $this->formId = $formId; } public function setFormId($formId) { $this->formId = $formId; } public function post($data) { $response = $this->httpClient->post( "https://docs.google.com/forms/d/$this->formId/formResponse", $this->makeGoogleFormData($data) ); return $response->getStatusCode() == 200; } private function makeGoogleFormData($data) { if ( ! isset($data['form_params'])) { $data = [ 'form_params' => $data ]; } return $data; } } </code></pre> <p>And a Laravel route using it:</p> <pre class="prettyprint"><code >Route::post('googleforms', function () { $googleForm = app()->make('GoogleForm', ['ABCDXRn7hdsLhrlDxalvDG6F4ISHQbT4duG2lZZl6OAHY']); $data = [ 'entry.123458658' => Input::get('name'), 'entry.123450697' => Input::get('city'), 'entry.123453423' => Input::get('school'), 'entry.1234596279' => Input::get('email'), 'entry.123452185' => Input::get('phone'), ]; return [ 'success' => $googleForm->post($data) ]; }); </code></pre> <p>Or can just require this <a href="https://github.com/antonioribeiro/googleforms">GoogleForms</a> package</p> <pre class="prettyprint"><code >composer require pragmarx/googleforms </code></pre> <p>Then use it</p> <pre class="prettyprint"><code >Route::post('googleforms', function () { $googleForm = app()->make('PragmaRX\GoogleForms\Client', ['ABCDXRn7hdsLhrlDxalvDG6F4ISHQbT4duG2lZZl6OAHY']); $data = [ 'entry.123458658' => Input::get('name'), 'entry.123450697' => Input::get('city'), 'entry.123453423' => Input::get('school'), 'entry.1234596279' => Input::get('email'), 'entry.123452185' => Input::get('phone'), ]; return [ 'success' => $googleForm->post($data) ]; }); </code></pre> <h3>Have fun!</h3>